Update Blueprint Function Library - BPFL_ShipSystem
parent
e50de61135
commit
59083b13d6
@ -11,3 +11,45 @@ This category of functions in the function library is used to retrieve variables
|
||||
This function takes a module class from the enumeration *E_ModuleClasses* and assigns a value to it for comparison. Since UE has issues comparing enums directly if they are not simply numerical this allows for you to define your classes with anything such as a phonetic alphabet like *Alpha, Bravo, Charlie...Zulu, etc.*. The scoring is done in reverse, so a lower score is a better class. Therefore *None* is assigned a high value like 500 and class one is given 1 while class 2 is given a score of 2 and so on. The letter ratings following a class A-D are given the same score as a decimal of the primary class. A is 1 (as it is the best rating/subclass it has the lowest value) and D is given 4. So class 3C is scored as 3.3.
|
||||
|
||||
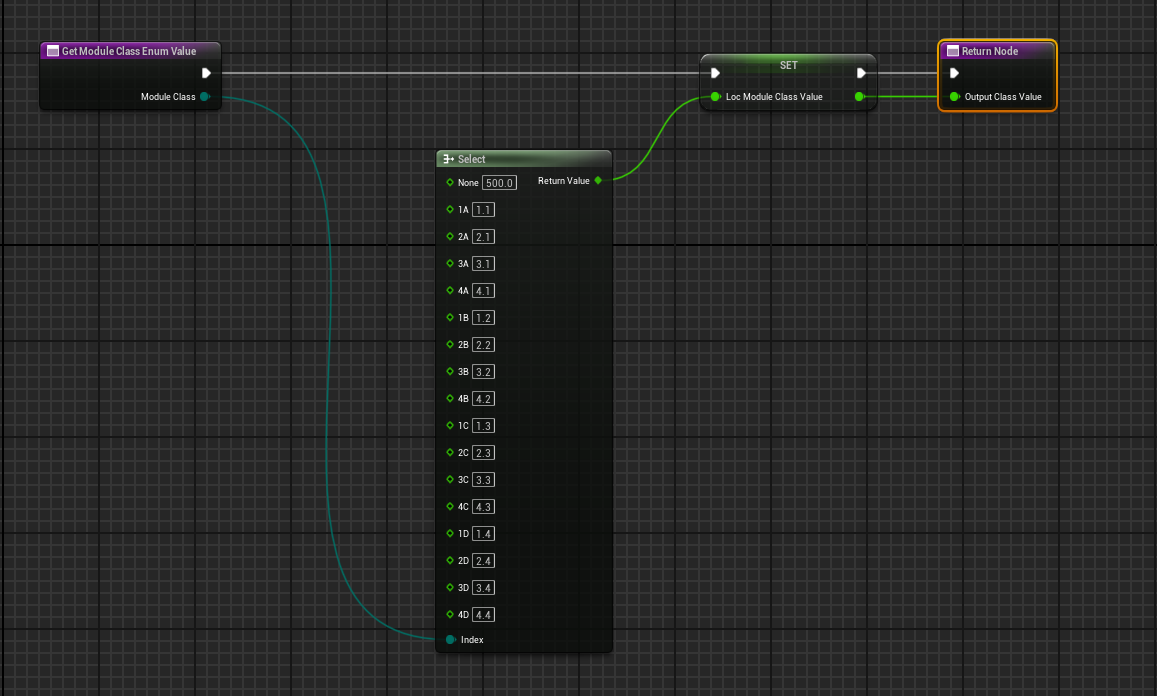
|
||||
|
||||
### GetPlayersCurrentlyEquippedModules
|
||||
|
||||
This function uses two local arrays to add items it finds as equipped to the player to the arrays returned by the function. It simply gets a list of all attached actors that are attached to the current player pawn, and then for each one of those attached actors it performs a *For Each Loop*. It then uses the Blueprint Interface Function, *Get Attached Actors and Sockets* to get the map variable, *AttachedActorsAndSockets* from the player pawn. This variable is documented later in the documentation but basically it stores a map of each actor attached to the player (even invisible or not visually altering actors) and their corresponding sockets (even non-existent sockets. Think of it more of an identifier of the 'slot' it is equipped to).
|
||||
|
||||
Using that retrieved Map Variable it finds a matching attached actor from the *For Each Loop* and if it finds a matching actor, it adds its actor object reference and matching socket to the local arrays respectively. After which it moves on to the next attached actor in the *For Each Loop*. When it has iterated over all actors it returns the Local Array variables via the functions return node.
|
||||
|
||||
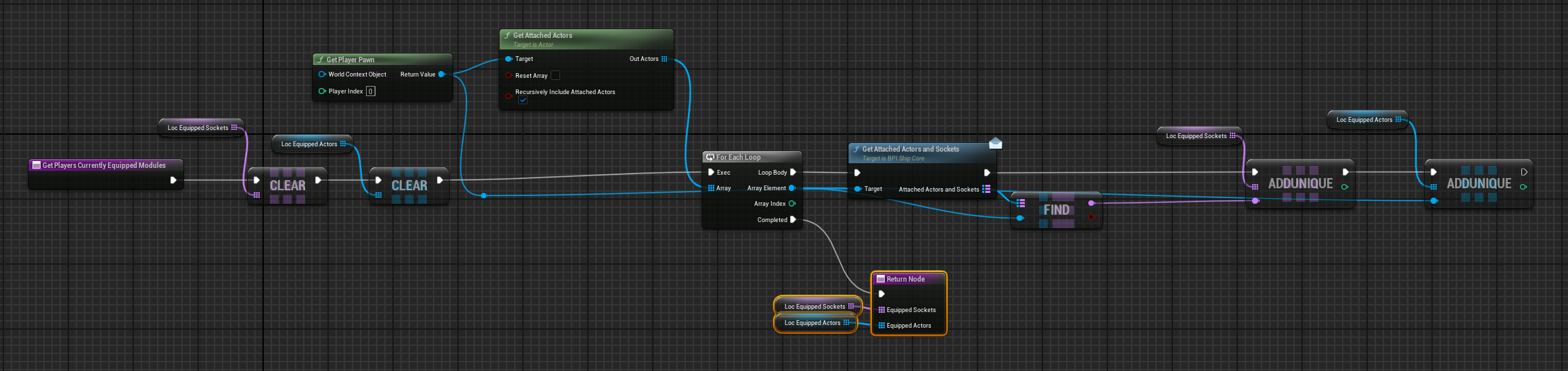
|
||||
|
||||
### GetEquippableModuleSlots
|
||||
|
||||
This function uses a local array to get all the Module loadout slots on the player. Configuring your pawns *Module Loadout* such as number of module slots, their class and so on is covered further in the documentation. The function uses the Blueprint Interface function *GetBaseShipInfo* which returns the struct *S_BaseShipInformation*. Within that struct we take the Map Variable *ModuleLoadout* and for each of its keys we enter a *For Each Loop*. Within that loop we check each of the keys to see if it contains the keyword *'Module'* and if it does we add it to the local array of Module Slots. When we are done iterating over the keys we return the local Module Slots array *LocModuleKeys* via the return node.
|
||||
|
||||
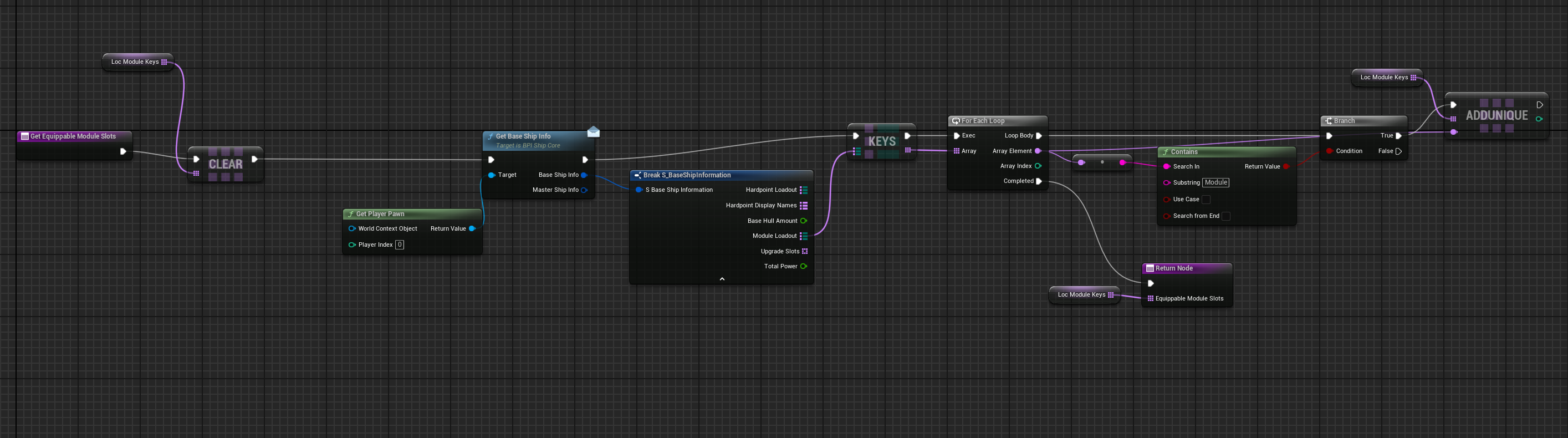
|
||||
|
||||
### GetUpgradeSlots
|
||||
|
||||
This function is very simple and used to cut down on a commonly used piece of blueprint code. It simply uses the *GetBaseShipInfo* Blueprint Function to retrieve the *UpgradeSlots* array variable and return it via the return node.
|
||||
|
||||
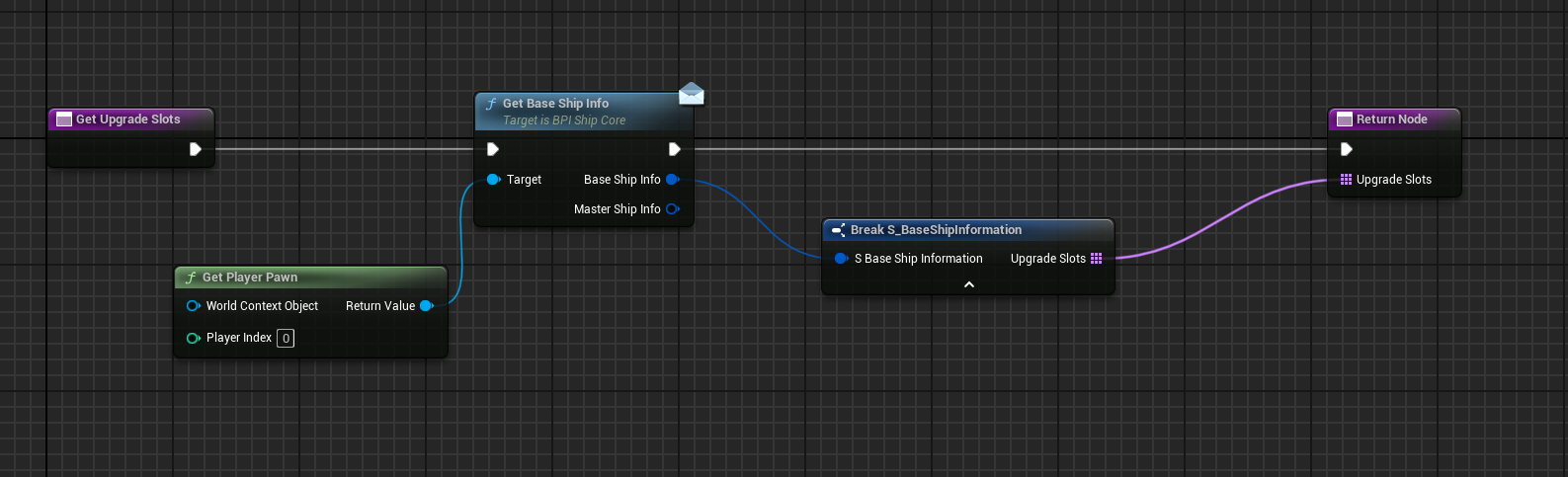
|
||||
|
||||
## Checkers
|
||||
|
||||
This category is different from *Getters* even though they technically are functions that *Get* something. These functions generally use the information they *Get* to return a Boolean or comparison.
|
||||
|
||||
### CompareModuleClassValues
|
||||
|
||||
This function uses the *Getter* function *GetModuleClassEnumValue* twice to take two module classes, A and B, and compare their corresponding scores. If module A is less than or equal to module B's score it will be True, otherwise False. The return node passes along this boolean.
|
||||
|
||||
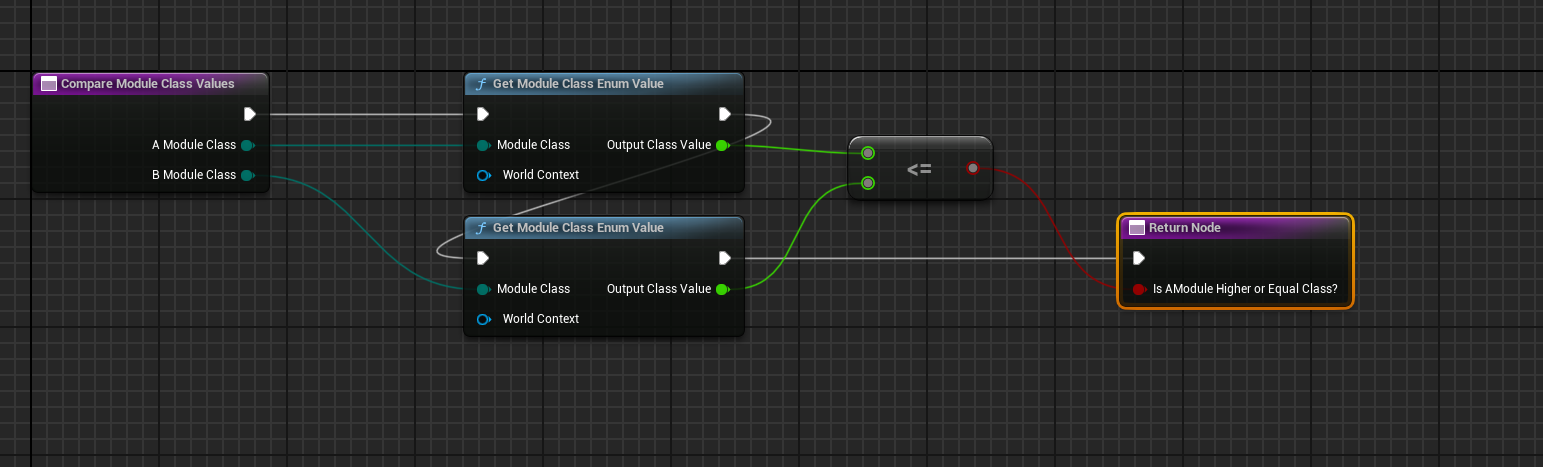
|
||||
|
||||
### CheckIfSlotHasItemEquipped
|
||||
|
||||
This function takes all of the player pawns attached actors and iterates over them in a *For Each Loop*. It also uses the *GetAttachedActorsAndSockets* Blueprint Function and its returned Map Variable. Within the *For Each Loop* it performs a *Find* on the *Attached Actors and Sockets* Map Variable to find if that map contains a matching actor to one that is attached to the player pawn. If there is a match it takes the value associated with that actor key and compares it to the input *Socket Name* from the function. If the value and the incoming socket name do not match it means the socket you are checking is not occupied even if an actor of the same name is already attached to the player pawn, as is the case if it was equipped to a different socket. If it is True, it means the socket is occupied with an actor as the one you are trying to check matches one found in the Map Variable. It then returns sets a local boolean to true and when the *For Each Loop* is finished it returns that boolean as well as the matching actor object reference. Note that if the function does not find any matching socket as having anything equipped, this return value *Equipped Actor* will be null/not valid.
|
||||
|
||||
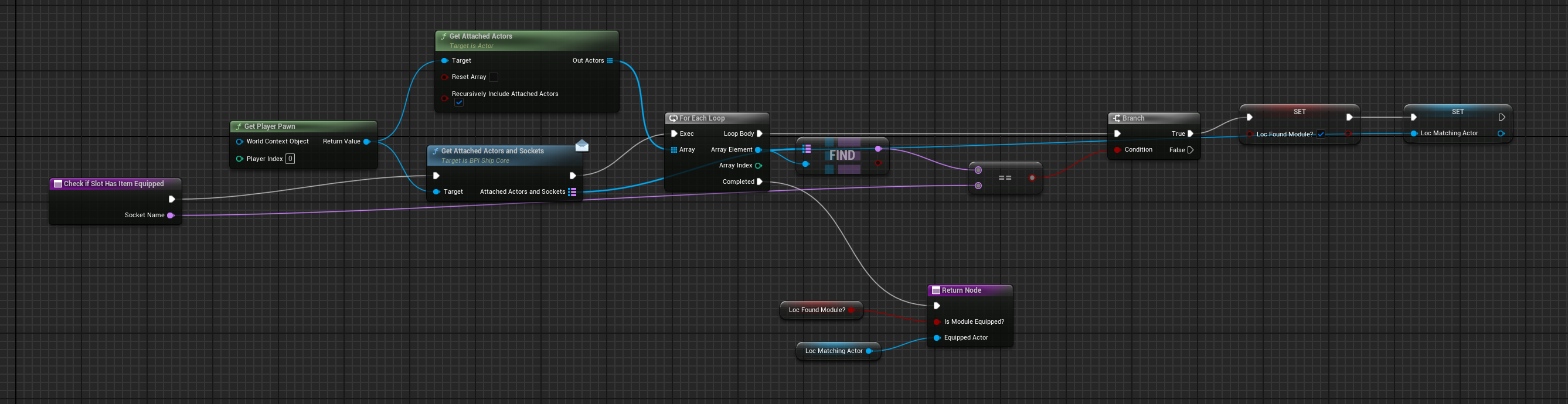
|
||||
|
||||
### CheckIfModuleTypeLimitExceeded?
|
||||
|
||||
This function can be used to check if a specific type of module, e.g. *Engine*, already has one equipped. This can be useful if you want to limit the number of a specific module the player can equip to their ship. In the demo files and by default in the kit, the only module that uses this is *Engine*. This function gets the player pawn's attached actors and the *AttachedActorsAndSockets* map variable as it does in the previous 2 *Checker* functions and iterates over them in a *For Each Loop* as well. Within the loop it uses the Blueprint Interface function *GetAttachedActorVariables* to retrieve information about each attached actor, specifically the module category of the attached actor. It then compares that enumeration to the input of the function, and if they match it sets a local boolean to true. When the loop is finished it returns the local boolean value, which by default is false. This will tell you if a module of the incoming category is already equipped to the player.
|
||||
|
||||
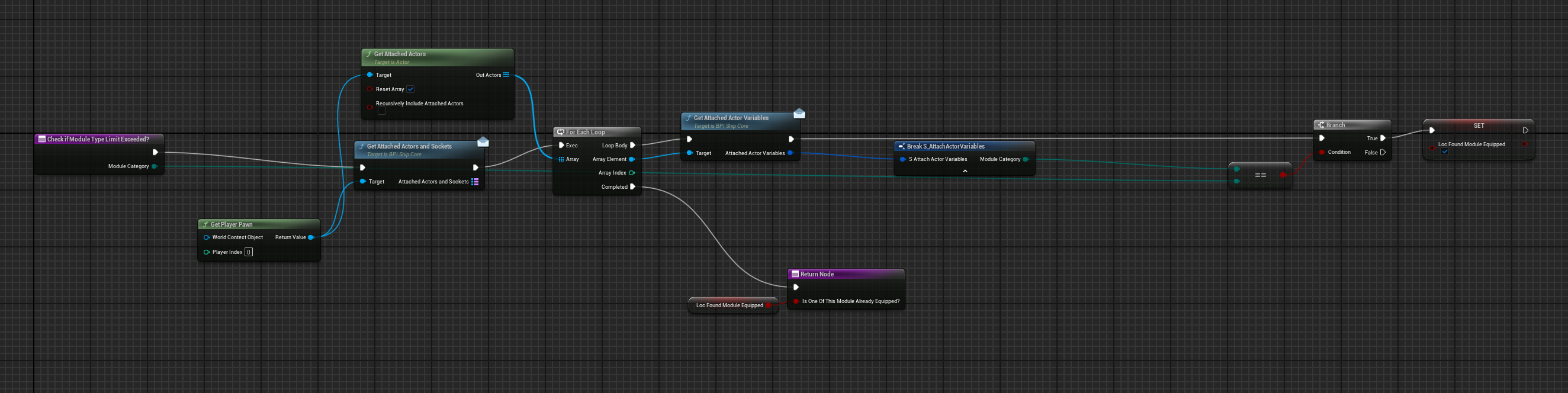
|
Loading…
Reference in New Issue
Block a user